24 July 2023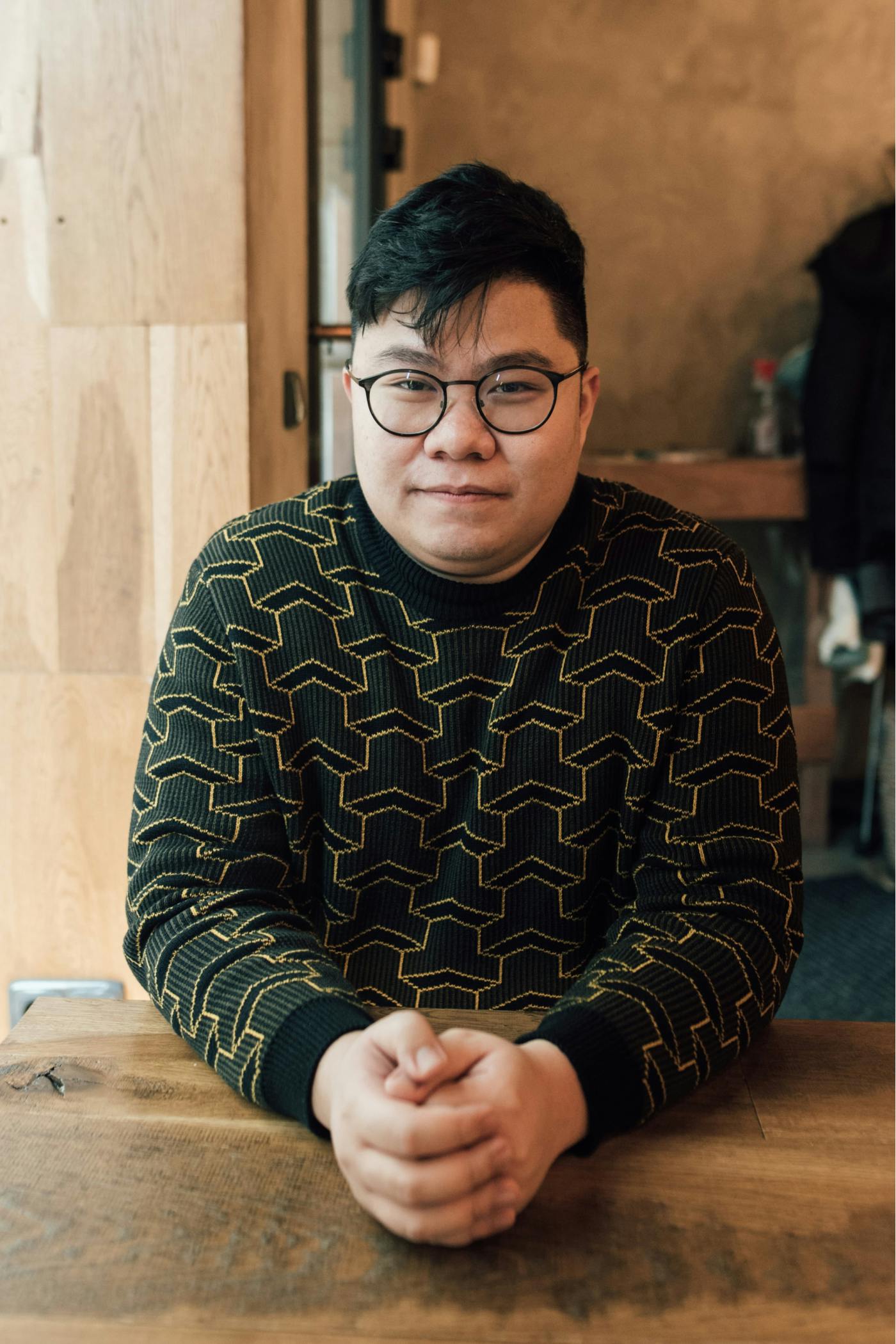
by
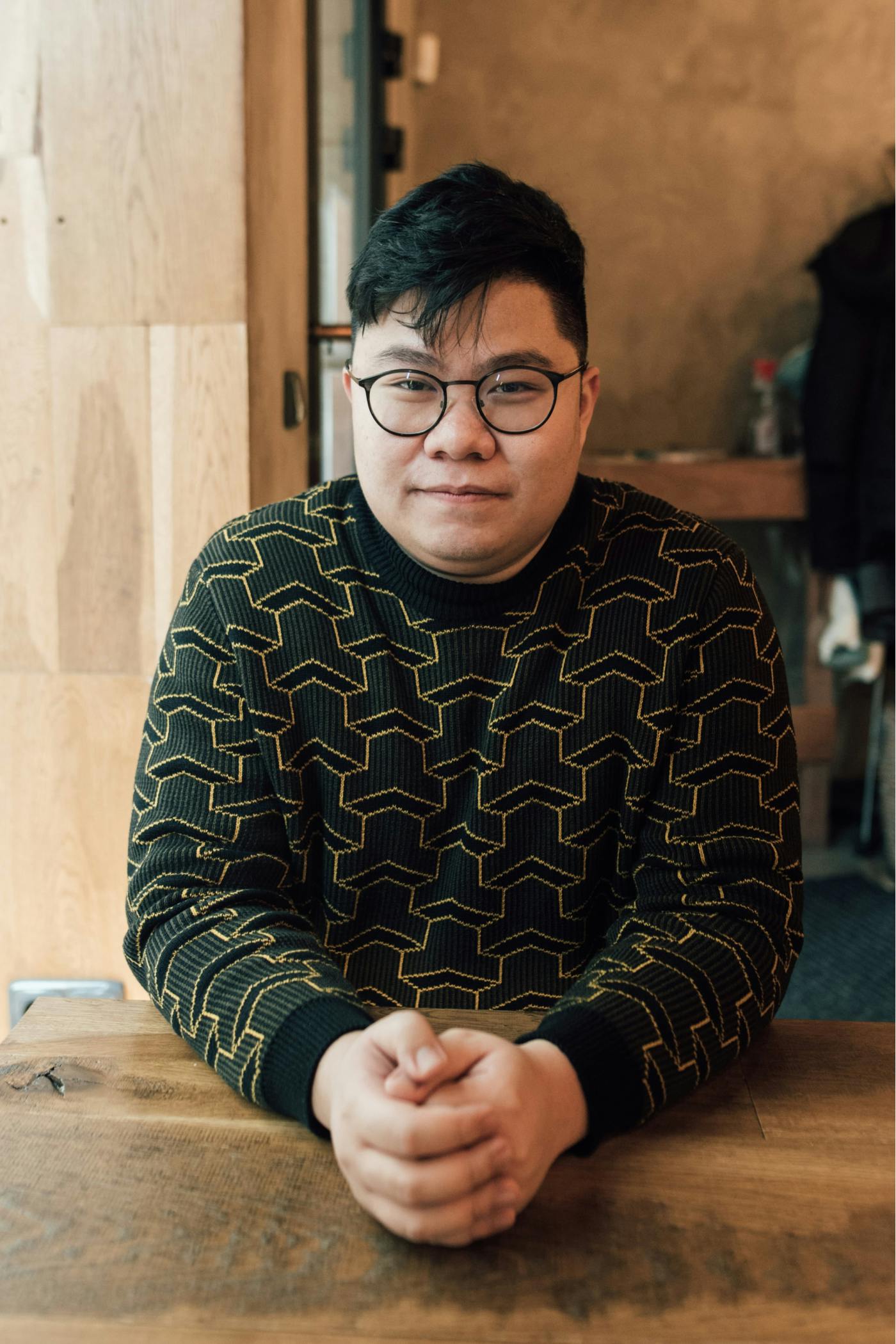
Tran Manh Hung
Unraveling the Magic of .env Files: Your Friendly Guide to Custom Variables in Next.js
custom variables
next.js
react
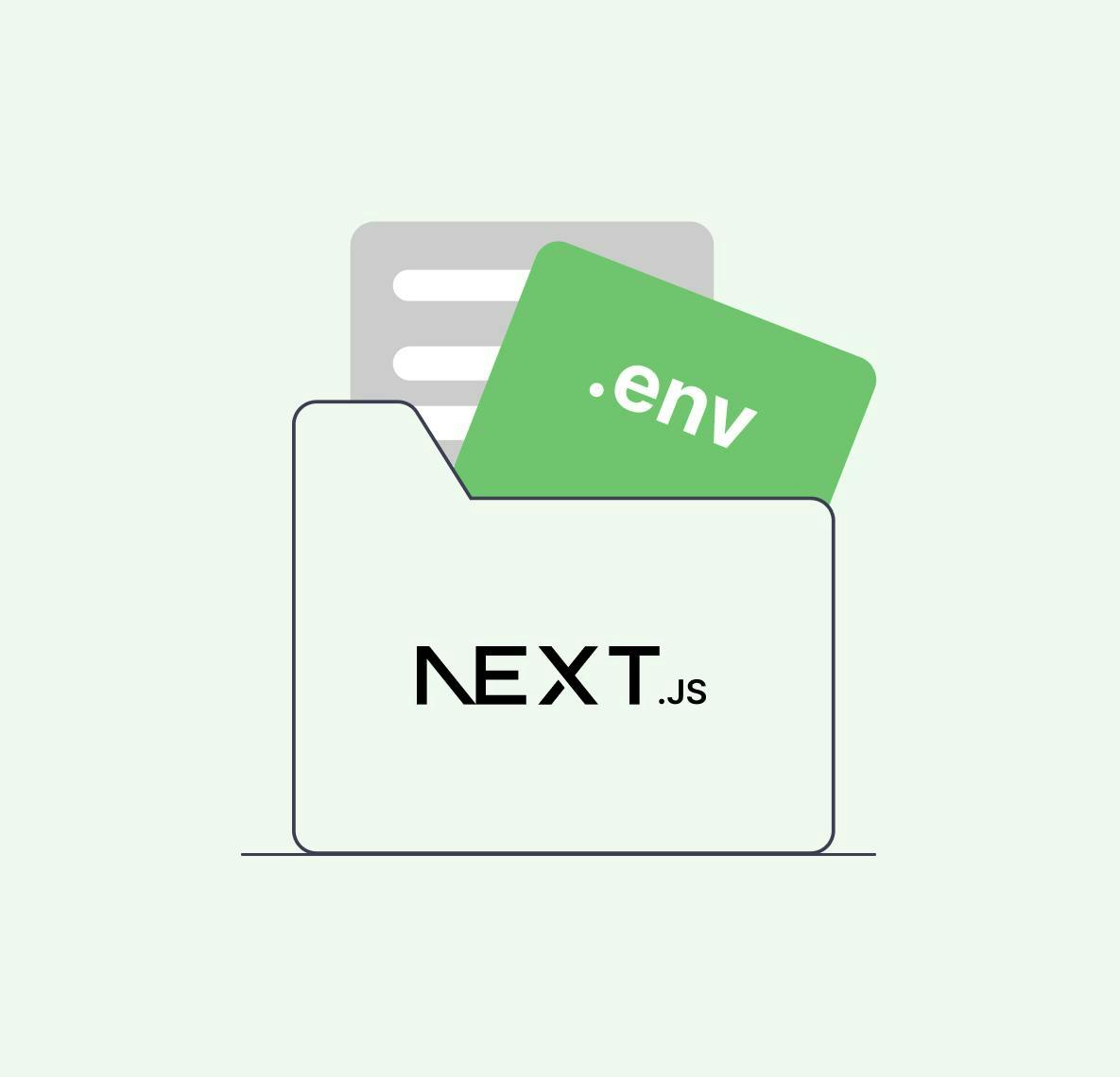
Have you ever found yourself in a situation where, amidst coding enthusiasm and focus, you accidentally committed an API key or secret onto GitHub? Most developers can relate to this scenario. Thankfully, ".env" files in Next.js React can save us from such blunders.
Introducing the .env File: Your Secret Keeper
Think of .env files as hidden vaults, safeguarding your top-secret info like API keys and database credentials. They protect your data from sneaky commit leaks on GitHub.
The Rules of .env Files in Next.js
- Your .env file's hideout should be at the root of your project. Don't try to place them elsewhere; they're crafty and won't be found.
- Next.js has different costumes for the .env files based on their environment:
.env
: Standard attire for all environments..env.local
: Local disguise. Seen in all environments except 'test.'.env.development
,.env.test
,.env.production
: Specific attire for each environment..env.development.local
,.env.test.local
,.env.production.local
: Local disguise for specific environments.
- Name your variables starting with the
REACT_APP_
prefix for the mission.
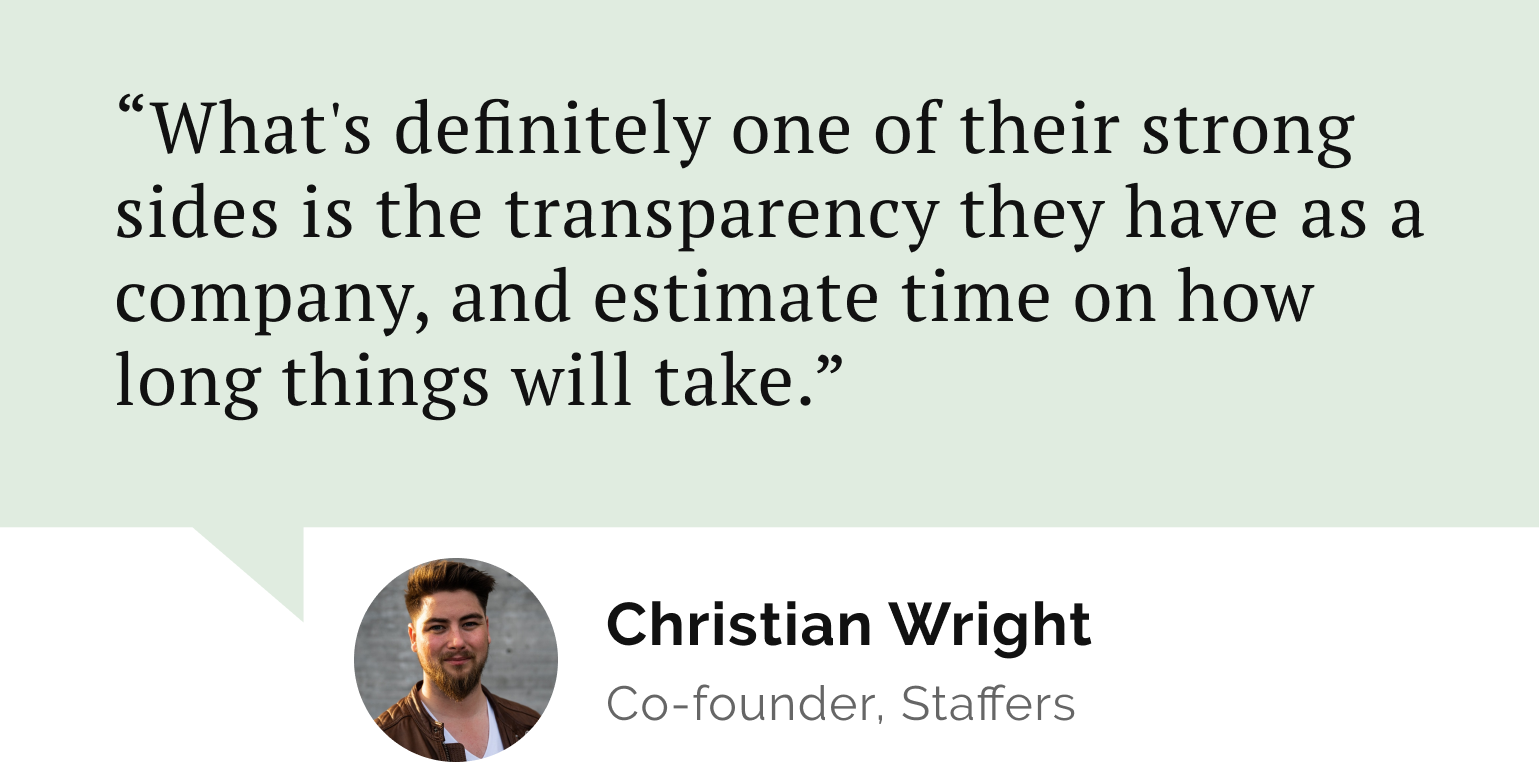
Do you need a reliable partner in tech for your next project?
Decoding the NEXT_PUBLIC_ prefix
When using Next.js, variables can only be accessed in the Node.js environment unless you include a prefix of either
NEXT_PUBLIC_
or REACT_APP_
. Doing so lets you regulate which data remains on the server side and which is sent to the browser.Here's an illustration:
1# .env file
2API_KEY_SERVER=12345678 # Only Node.js can see this
3NEXT_PUBLIC_API_KEY_CLIENT=87654321 # Node.js and the browser can see this
Deploying Your Secret Variables
Once you've given your variables their correct aliases, you can deploy them using the
process.env
gadget provided by Node.js. Here's an example:You have these defined in your .env file:
1# .env file
2REACT_APP_API_URL=https://api.mywebsite.com
3NEXT_PUBLIC_SITE_NAME=MyCoolWebsite
You can then deploy these in your React components:
1// SomeReactComponent.js
2import React from 'react';
3
4const SomeReactComponent = () => {
5 return (
6 <div>
7 <h1>Welcome to {process.env.NEXT_PUBLIC_SITE_NAME}!</h1>
8 <p>We fetch data from: {process.env.REACT_APP_API_URL}</p>
9 </div>
10 );
11};
12
13export default SomeReactComponent;
Custom TypeScript Script and .env.local
In Next.js, the
.env.local
file is meant to store environment variables that are specific to your local development environment. When you run next dev, Next.js automatically loads the environment variables from .env.local
and makes them available in your application.However, suppose you are trying to access these environment variables from a custom TypeScript script (outside your Next.js application). In that case, it won't be able to read them directly because the next CLI is not involved in running the custom script. The custom TypeScript script is a separate entity unaware of Next.js' environment variables.
Suppose you want your custom TypeScript script to access the environment variables from
.env.local
. In that case, you can use a package like dotenv, which loads the variables from the .env.local
file and makes them available to your script.Here's how you can do it:
Step 1: Install the dotenv package:
1npm install dotenv --save
Step 2: Create a dotenv configuration file in your project (e.g., dotenv-config.ts) and add the following code:
1import dotenv from 'dotenv';
2
3// Load environment variables from .env.local file
4dotenv.config({ path: '.env.local' });
5
6// Now you can access the environment variables using process.env
7const myVariable = process.env.MY_VARIABLE;
Conclusion
These variables will transform into their actual values at build time. They offer flexible disguise options for different environments like development, testing, and production.
So, there you have it—an easy, secure, and flexible way to manage your secrets in Next.js React. Keep your secrets safe and happy coding.
Let’s stay connected
Do you want the latest and greatest from our blog straight to your inbox? Chuck us your email address and get informed.
You can unsubscribe any time. For more details, review our privacy policy