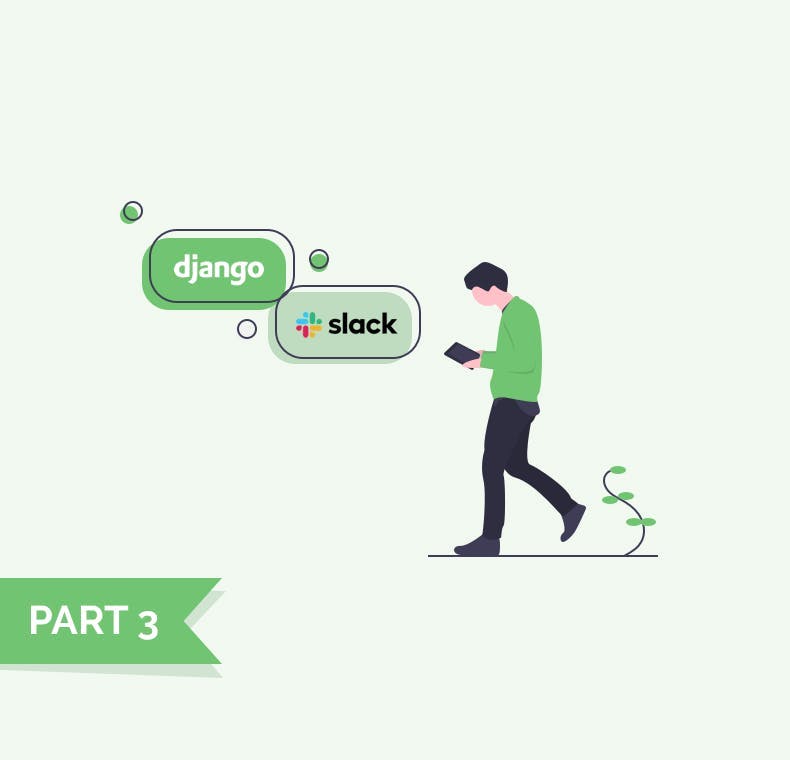
In order to interact with Slack, we need to quickly build some business logic.
Luckily, that is super easy with Django. We will generate some entities using Django models, and provide an admin interface so that we can create & modify them. One of the reasons I've chosen Django for this tutorial is that we can do this without building a custom UIs.
Our application will allow users to log in and report hours on their projects. They'll also be able to see other user's reports. Simple as that.
We will:
- Create
Project
model that will hold information about a project ProjectReport
model that will be used to report hours for each project
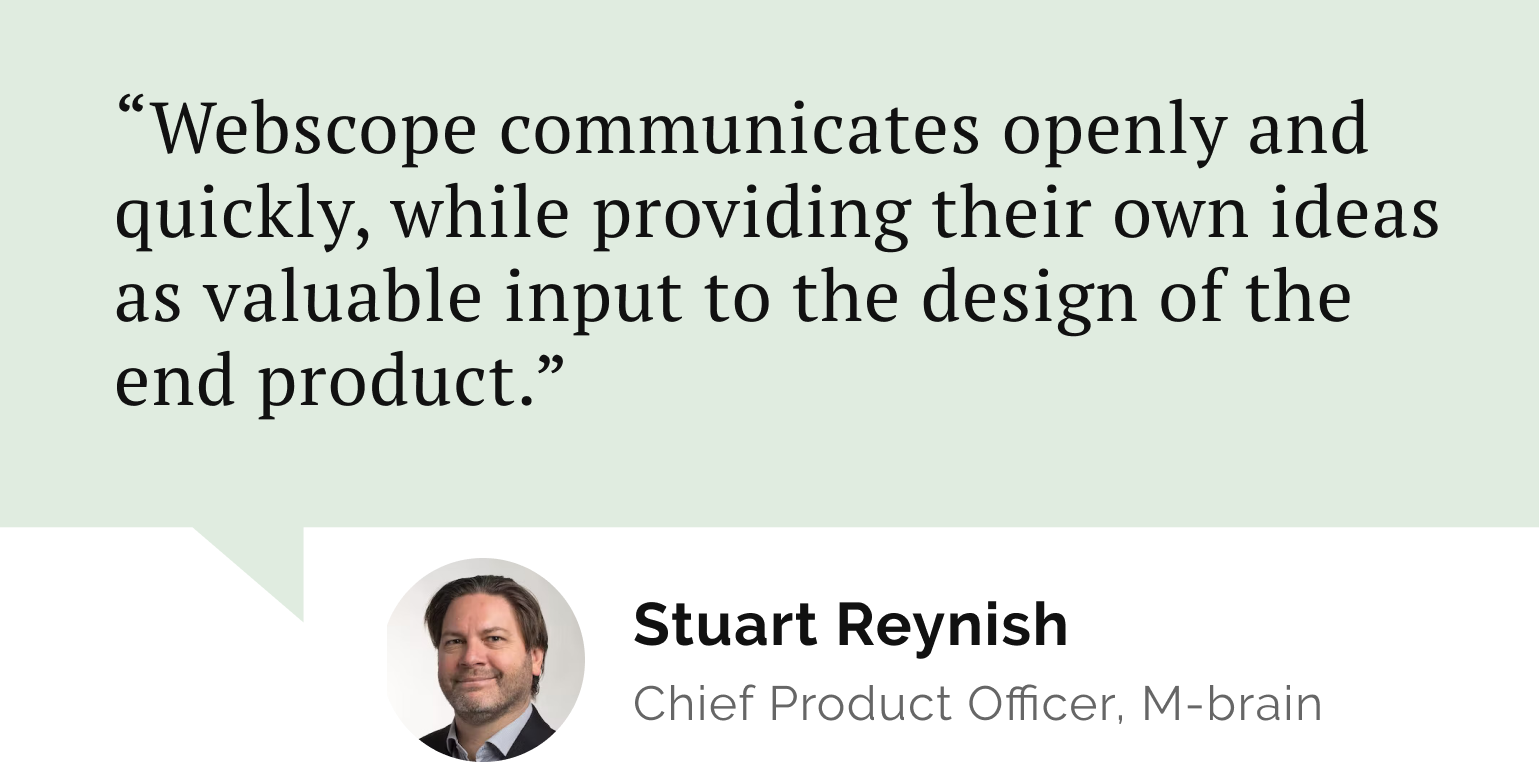
Do you need a reliable partner in tech for your next project?
Create reports models
pipenv run ./manage.py startapp projects
- Add
projects
string toINSTALLED_APPS
in yoursettings.py
- Create
Project
andProjectReport
model
projects/models.py
1from django.contrib.auth import get_user_model
2from django.db import models
3
4User = get_user_model()
5
6class Project(models.Model):
7 name = models.CharField(max_length=255)
8 logo = models.ImageField(null=True, blank=True)
9
10 def __str__(self):
11 return self.name
12
13
14class ProjectReport(models.Model):
15 project = models.ForeignKey(Project, on_delete=models.CASCADE)
16 description = models.TextField()
17 hours = models.FloatField()
18 # if we delete a user we don't want to loose the reported hours
19 user = models.ForeignKey(User, on_delete=models.PROTECT)
20
21 def __str__(self):
22 return f"{self.project.name} - {self.hours} hours - {self.user.username}"
and add them to admin interface
projects/admin.py
1from django.contrib import admin
2
3from .models import Project, ProjectReport
4
5admin.site.register(Project)
6admin.site.register(ProjectReport)
pipenv install Pillow
We need to do this, because we've added an ImageField..
pipenv run ./manage.py makemigrations
&&pipenv run ./manage.py migrate
git push
deploy master
from Heroku.🎉 You can now check our models in the admin interface.
Let’s stay connected
Do you want the latest and greatest from our blog straight to your inbox? Chuck us your email address and get informed.
You can unsubscribe any time. For more details, review our privacy policy